Tools For Web Developers Introduction
Table of Contents
In the demanding world of web development, having the right tools at your disposal can make all the difference in the world. The wide range of tools available today are designed to boost productivity, streamline workflows, and improve code quality. A well-curated toolkit allows developers to focus on creating innovative, high-quality web solutions while working more efficiently.
Productivity is a crucial aspect for web developers, as it directly impacts project timelines and the ability to meet deadlines. The right tools can automate repetitive tasks, facilitate error detection, and provide integrated development environments that support seamless coding experiences. Streamlined workflows, achieved through the use of these tools, ensure that developers can manage their projects effectively, collaborate with team members, and maintain clear and organized codebases.
Moreover, the quality of code is paramount in web development. Tools that assist in code validation, debugging, and optimization play a vital role in maintaining high standards. These tools not only help in identifying and rectifying errors early in the development process but also ensure that the final product is robust, secure, and performs optimally across different platforms and devices.
This blog post will delve into 10 essential tools that every web developer should consider incorporating into their workflow. Each tool has been selected based on its ability to address specific needs within the development process, from coding and debugging to design and deployment. By integrating these tools, developers can enhance their productivity, streamline their workflows, and ultimately produce higher-quality code.
1. Code Editors
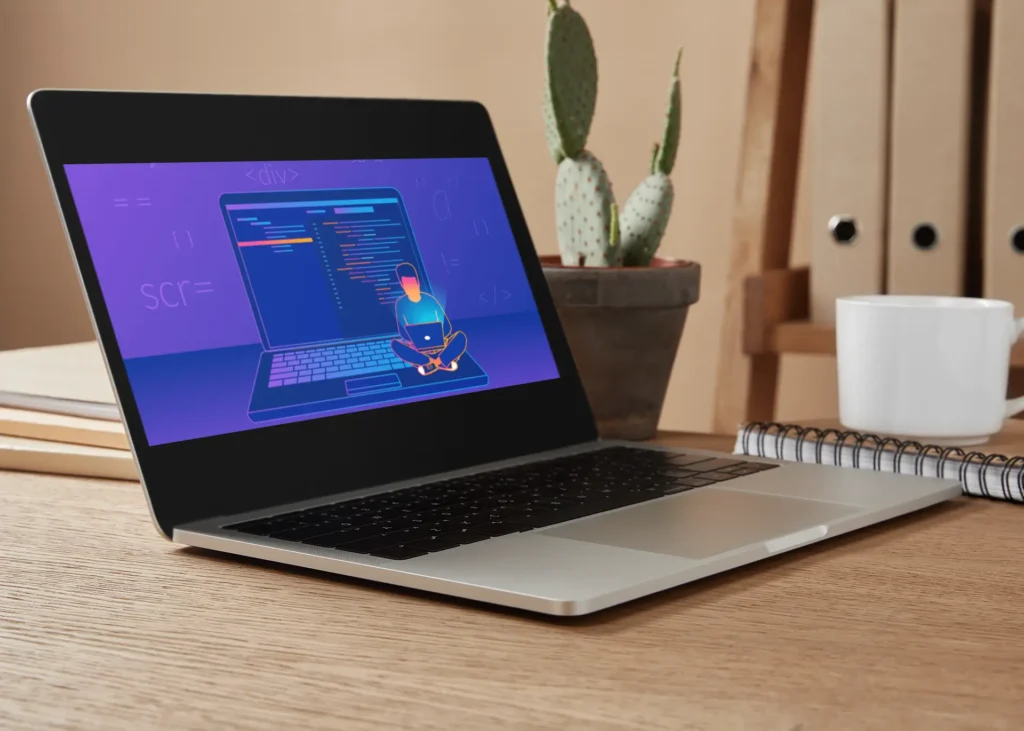
For web developers, having a powerful code editor is indispensable in crafting clean, efficient, and bug-free code. Code editors streamline the development process by offering a range of features designed to enhance productivity and accuracy. Popular options in the realm of code editors include Visual Studio Code, Sublime Text, and Atom, each bringing unique strengths to the table.
Visual Studio Code, often abbreviated as VS Code, stands out with its rich feature set. It offers an extensive library of extensions, integrated Git commands, and an intuitive user interface. Syntax highlighting and code completion are built-in, reducing the time developers spend on identifying errors and improving code readability. Additionally, VS Code’s customizable interface allows developers to tailor the editor to their specific needs, making it a versatile choice for various programming languages. For a detailed review of Visual Studio Code, you can refer to our comprehensive overview here. To explore further, visit the official VS Code website.
Sublime Text is another noteworthy code editor known for its speed and simplicity. It supports a wide range of programming languages and offers features such as split editing, distraction-free mode, and a powerful search functionality. Sublime Text’s lightweight design ensures that it runs smoothly even on less powerful machines, making it a favorite among developers who value performance. For an in-depth look at Sublime Text, check out our detailed analysis here. More information is available on the official Sublime Text website.
Atom, developed by GitHub, is a highly customizable code editor that integrates seamlessly with Git and GitHub. It offers a built-in package manager, smart autocompletion, and a file system browser. Atom’s hackable nature allows developers to modify the editor to suit their workflow perfectly. If you’re interested in learning more about Atom, you can read our full review here. Visit the official Atom website for additional details.
In essence, selecting the right code editor can significantly impact your coding efficiency and overall development experience. By leveraging the robust features offered by these top code editors, web developers can enhance their productivity and achieve greater success in their projects.
Version Control Systems
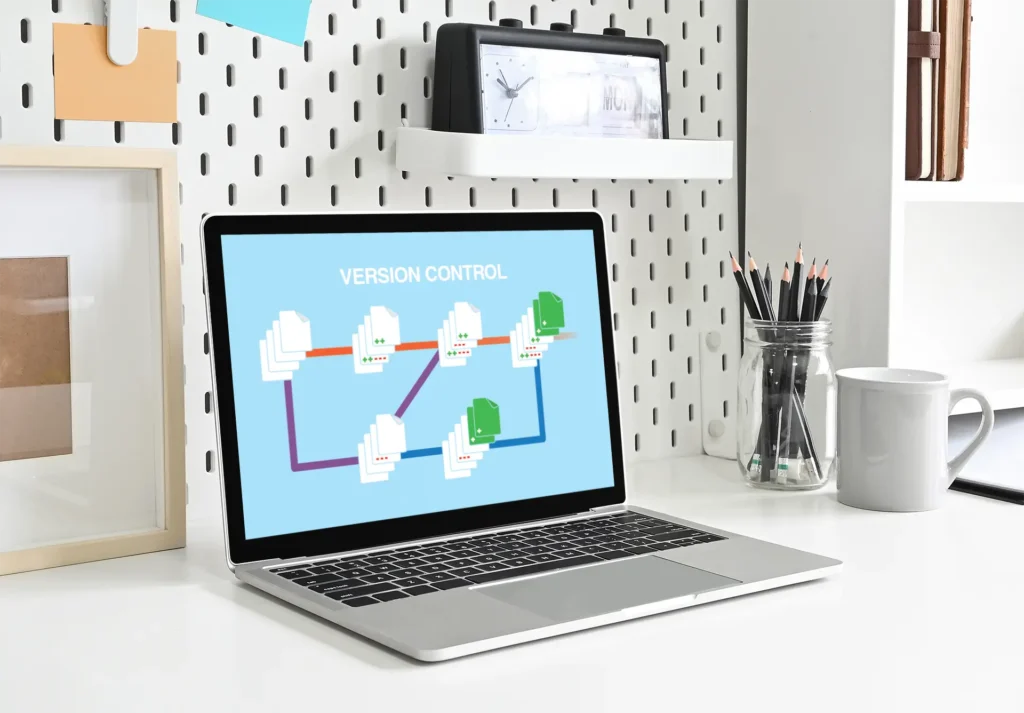
Version control systems (VCS) are indispensable tools for web developers, providing a structured way to manage and track changes in codebases. They offer a collaborative environment where multiple developers can work on the same project without overwriting each other’s changes. Among the various VCS available, Git and GitHub stand out as essential tools for modern web development.
Git, a distributed version control system, allows developers to track changes, revert to previous states, and branch off to work on new features without impacting the main codebase. This flexibility is crucial for maintaining the integrity and stability of a project. Git stores snapshots of your project, enabling you to explore the history of your code and understand the evolution of your project over time.
GitHub, a cloud-based platform built around Git, enhances these capabilities by providing a collaborative space for sharing and managing repositories. It facilitates code reviews, issue tracking, and project management, making it easier for teams to coordinate their efforts. GitHub’s pull request feature is particularly valuable, allowing developers to propose changes, discuss modifications, and merge updates seamlessly.
For effective use of Git and GitHub, consider the following best practices:
- Commit Frequently: Regular commits with clear messages help track progress and make it easier to identify and fix issues.
- Branch Strategically: Use branches to work on features or fixes independently from the main codebase. This approach minimizes conflicts and ensures the stability of the main branch.
- Merge Thoughtfully: Regularly merge changes from feature branches into the main branch, and resolve conflicts early to maintain a clean and functional codebase.
- Review Code: Utilize GitHub’s pull request feature for thorough code reviews, ensuring quality and consistency across the project.
For a deeper understanding of version control, refer to our detailed tutorials on version control. Additionally, GitHub’s official documentation offers comprehensive guidance on utilizing its features effectively.
Package Managers
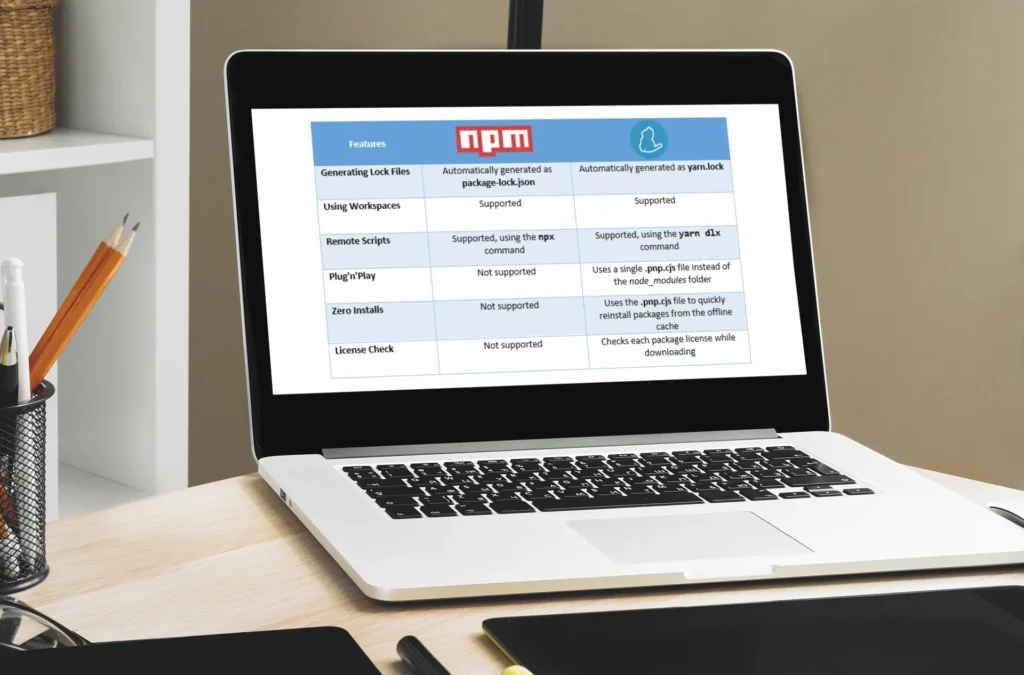
Package managers such as npm and Yarn are indispensable tools for web developers, facilitating the management of project dependencies. These tools simplify the often complex process of installing, updating, and managing libraries and frameworks, ensuring that projects remain consistent and up-to-date. npm (Node Package Manager) is one of the most widely used package managers, particularly in the JavaScript ecosystem. It allows developers to easily incorporate third-party packages into their projects and manage their versions efficiently.
Yarn, another prominent package manager, offers similar functionalities but is known for its speed and reliability. Yarn uses a lock file to ensure that installations are deterministic and consistent across different environments, which can be particularly beneficial for larger projects. Both npm and Yarn support a variety of common commands that streamline dependency management.
For instance, the command npm install
or yarn add
can be used to add a new package to a project. To remove a package, one would use npm uninstall
or yarn remove
. Updating packages is straightforward with npm update
or yarn upgrade
. These commands are essential for maintaining a healthy project environment.
Use cases for package managers extend beyond just adding and removing dependencies. They also enable developers to create scripts for automating various tasks, such as running tests or building the project. This can be achieved with the npm run
or yarn run
commands, which execute scripts defined in the project’s configuration file.
For those new to these tools, our internal guides on getting started with npm provide a comprehensive introduction. Additionally, the official documentation for npm and Yarn offers extensive resources and best practices for effective package management.
CSS Preprocessors
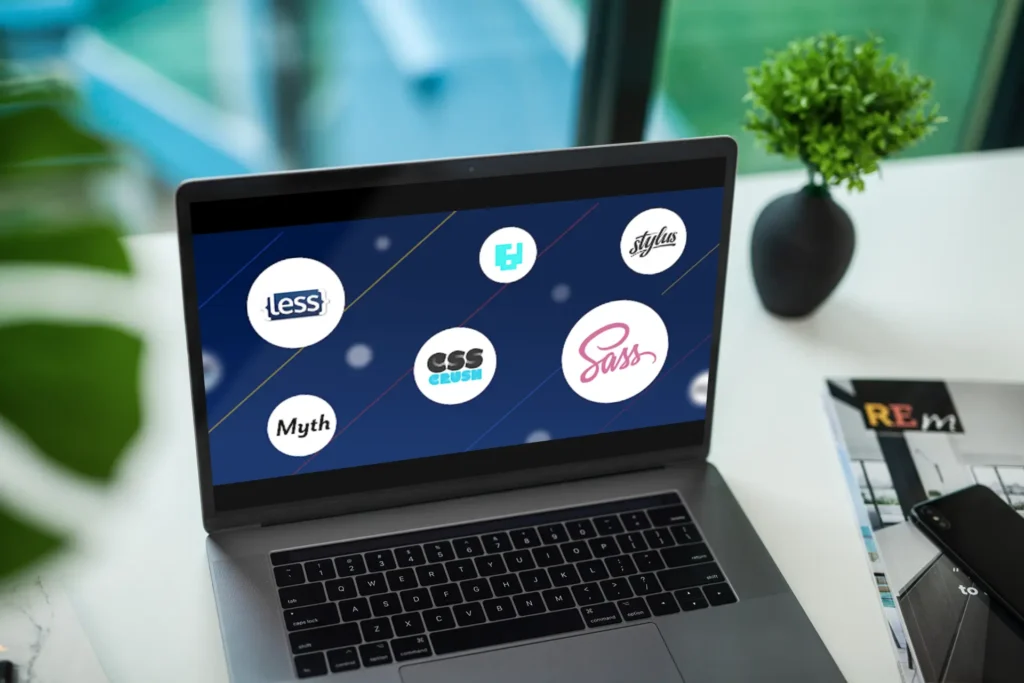
CSS preprocessors have become indispensable tools for web developers aiming to write maintainable and scalable CSS. Among the most popular are Sass and LESS, both of which offer a range of features that streamline the styling process significantly. By utilizing CSS preprocessors, developers can take advantage of advanced functionalities such as variables, nesting, and mixins, making their CSS more organized and easier to manage.
Variables in CSS preprocessors allow developers to store values that can be reused throughout the stylesheet. For example, instead of repeatedly typing a specific color code, you can define it once and reference it multiple times. This not only saves time but also ensures consistency across the project. For instance, in Sass, you can define a variable like $primary-color: #3498db;
and use color: $primary-color;
wherever needed.
Nesting is another powerful feature that helps in writing cleaner and more readable CSS. It allows you to nest your CSS selectors in a way that follows the same visual hierarchy of your HTML. This results in more structured and readable code. For example, in Sass, you can write:
nav {ul {margin: 0;padding: 0;list-style: none;}li { display: inline-block; }a {display: block;padding: 6px 12px;text-decoration: none;}}
Mixins are another standout feature of CSS preprocessors. They allow you to create reusable chunks of code that can be included in other styles. This is particularly useful for applying common patterns or vendor prefixes. For instance, a mixin in Sass can be written as follows:
@mixin border-radius($radius) {-webkit-border-radius: $radius;-moz-border-radius: $radius;border-radius: $radius;}
You can then include this mixin in your CSS rules like so: @include border-radius(10px);
For more detailed information, you can explore our in-depth posts on Sass and LESS. Additionally, visit the official sites of Sass and LESS to dive deeper into their documentation and features.
5. Task Runners
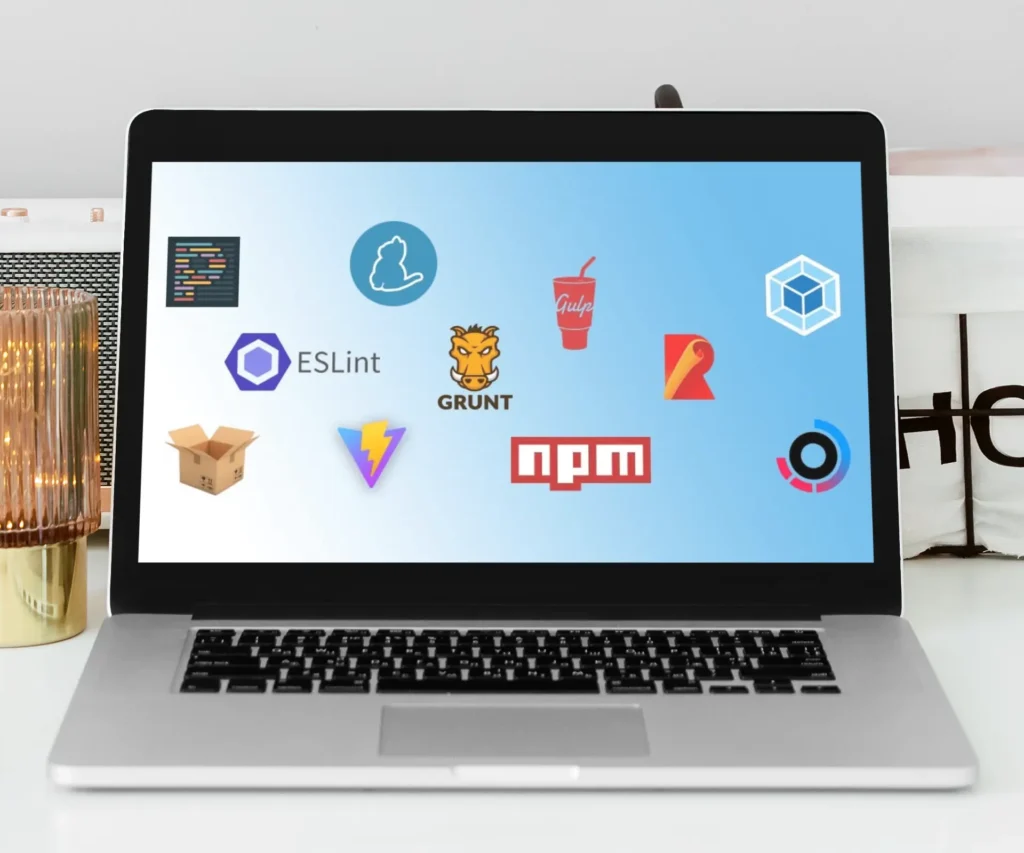
Task runners, such as Gulp and Grunt, are indispensable tools for web developers. They streamline workflow by automating repetitive tasks, thereby increasing efficiency and reducing the potential for human error. Task runners can handle a variety of tasks, including minification, compilation, and testing, among others.
Gulp, known for its simplicity and speed, allows developers to create tasks using a series of plugins and a straightforward API. For example, a typical Gulp task might involve minifying JavaScript files, compiling Sass into CSS, and automatically reloading the browser whenever changes are detected. Grunt, on the other hand, relies on configuration files to define tasks, making it highly customizable and versatile. It can perform similar functions to Gulp, such as image optimization, file concatenation, and unit testing.
Among the core features of task runners are their ability to handle file transformations and automate build processes. With these tools, developers can ensure that their code is consistently formatted, free of syntax errors, and optimized for performance. For instance, during the build process, a task runner might transpile ES6+ JavaScript into ES5 for broader browser compatibility, or compress image files to reduce load times.
Common tasks handled by Gulp and Grunt include:
- Minification of CSS, JavaScript, and HTML files to reduce file size and improve load times.
- Compilation of preprocessor languages like Sass, Less, and TypeScript into standard CSS and JavaScript.
- Linting code to catch syntax errors and enforce coding standards.
- Running unit tests to ensure code functionality and reliability.
- Watching files for changes and automatically performing tasks such as reloading the browser or re-running tests.
For those new to task runners, numerous tutorials are available to help with setup. For Gulp, our Gulp setup guide offers a step-by-step introduction. Similarly, our Grunt setup tutorial provides a comprehensive overview for beginners. For more detailed information, refer to the official documentation for Gulp and Grunt.
Frameworks and Libraries
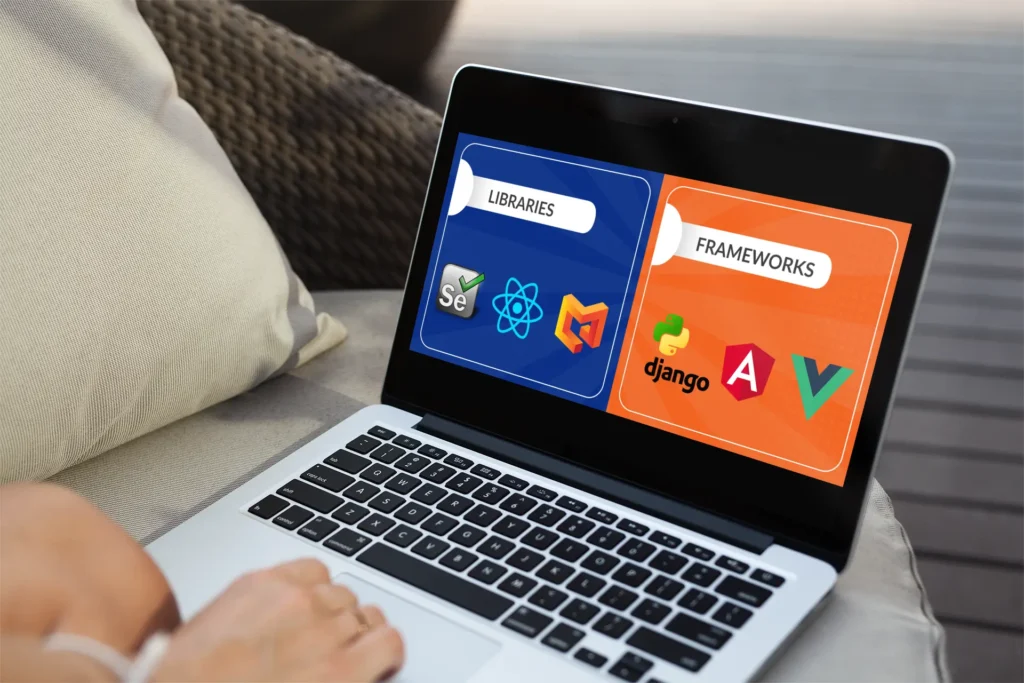
Frameworks and libraries play a crucial role in accelerating the web development process by providing pre-written code and standardized patterns. These tools streamline development, ensuring that developers can focus on building unique features rather than reinventing the wheel. Popular options such as React, Angular, and Vue.js are commonly employed by developers due to their robust capabilities and supportive communities.
React, developed by Facebook, is renowned for its efficiency in building user interfaces, particularly for single-page applications. React’s component-based architecture allows developers to create reusable UI components, which significantly enhances productivity. Additionally, its virtual DOM improves performance by minimizing direct manipulation of the real DOM. For more detailed comparisons, you can refer to our React vs. Angular vs. Vue.js article.
Angular, maintained by Google, is a comprehensive framework suitable for developing large-scale, enterprise-level applications. It offers a complete toolbox, including data binding, dependency injection, and a powerful CLI for streamlining development tasks. Angular’s two-way data binding feature ensures synchronization between the model and the view, simplifying the process of keeping the user interface in sync with the underlying data. To explore more about Angular, visit the official Angular documentation.
Vue.js, created by Evan You, is celebrated for its gentle learning curve and flexibility. Vue’s reactive data binding system and component-based structure make it a preferred choice for both small-scale and large-scale applications. Its lightweight nature and straightforward integration with other projects without extensive reconfiguration are key advantages. For comprehensive information, check out the official Vue.js guide.
By leveraging these frameworks and libraries, web developers can significantly reduce development time, enhance code quality, and improve application performance. Each tool has its unique features and ideal use cases, making them indispensable assets in the modern web development toolkit.
Development Environments
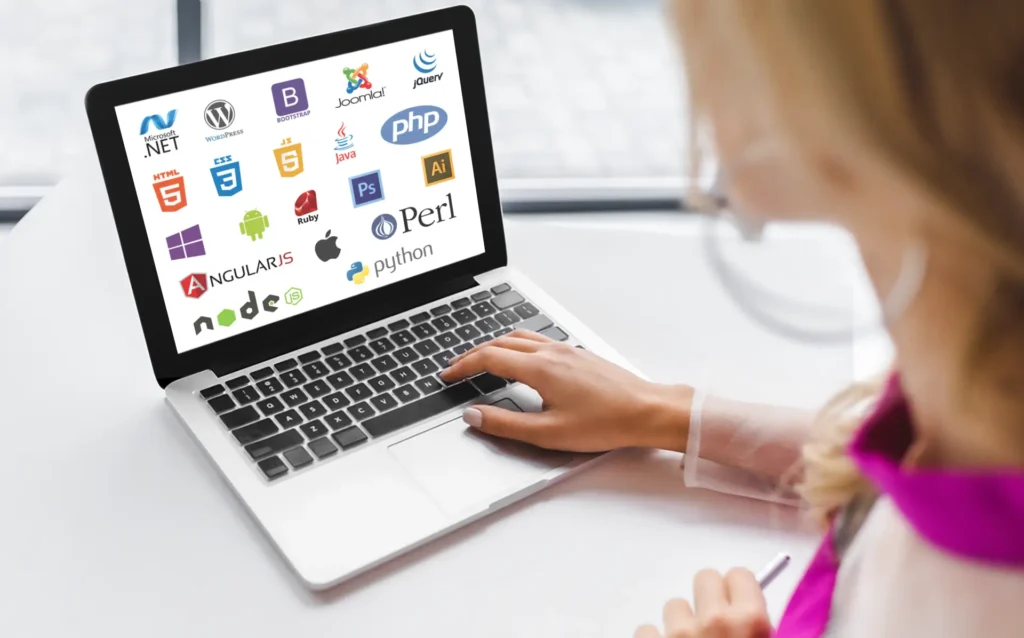
Integrated development environments (IDEs) are comprehensive tools that provide web developers with a suite of functionalities designed to streamline the coding process. Unlike basic code editors, IDEs such as WebStorm and Eclipse offer advanced features like built-in debugging, version control, and code refactoring, which significantly enhance productivity and code quality.
WebStorm, developed by JetBrains, is a powerful IDE tailored for JavaScript and web development. It supports modern frameworks such as React, Angular, and Vue.js, and offers intelligent coding assistance, including code completion, navigation, and on-the-fly error detection. Furthermore, WebStorm’s seamless integration with Git and other version control systems makes it easy to manage code changes and collaborate with team members. For a comprehensive review of WebStorm, you can check our detailed analysis here.
Similarly, Eclipse is another popular IDE that caters to a wide range of programming languages, including Java, C++, and PHP. Eclipse’s modular architecture allows developers to customize their environment with various plugins, enhancing its functionality. The IDE’s robust debugging tools and support for different build systems make it a versatile choice for many developers. For more insights into Eclipse, visit their official website.
When comparing IDEs, factors such as language support, ease of use, and community support play critical roles. While WebStorm excels in JavaScript development with its rich feature set and intuitive interface, Eclipse stands out for its flexibility and extensive plugin ecosystem. Ultimately, the choice of an IDE depends on individual project requirements and personal preferences.
For those interested in exploring other IDE options, our in-depth reviews of popular development environments can be found here. Each review highlights the ussssssssssssssssssssssssssssnique features and benefits of the IDE, helping you make an informed decision.
Testing Tools
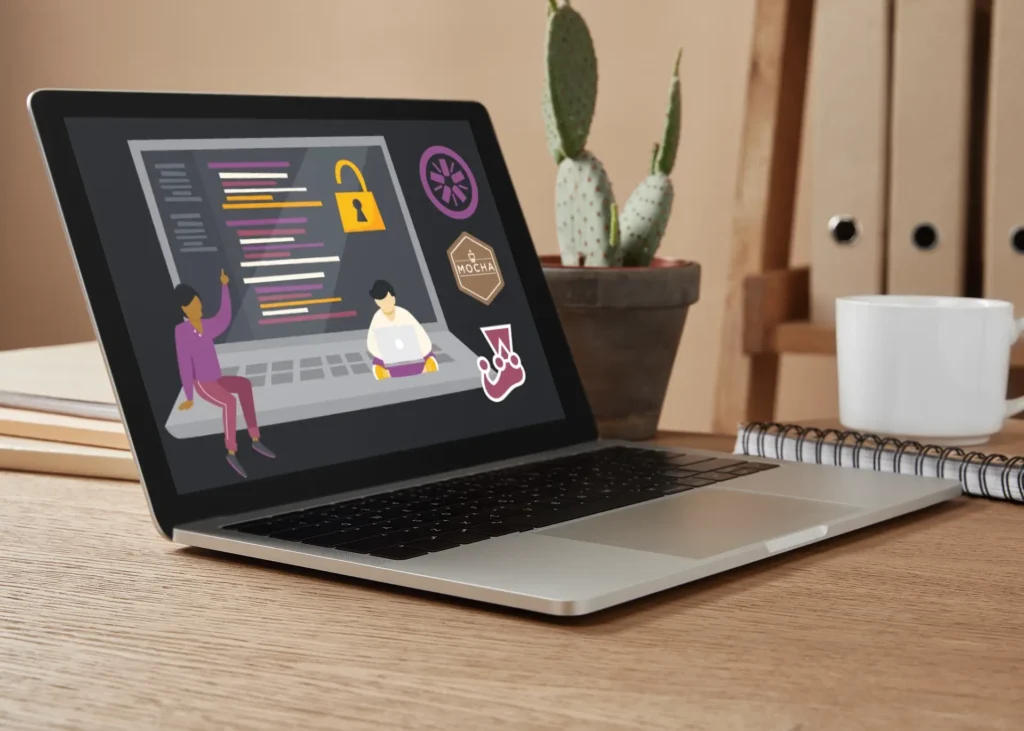
Testing is a fundamental aspect of web development that ensures the reliability, performance, and security of web applications. Comprehensive testing can identify bugs early, enhance user experience, and maintain high standards of code quality. There are several types of testing, each serving a unique purpose in the development lifecycle.
Unit Testing focuses on individual components or functions. Tools like Jest and Mocha are popular for this purpose. Jest, created by Facebook, is particularly favored for its simplicity and efficiency, especially in React applications. Mocha, known for its flexibility, works well with other libraries such as Chai for assertions.
Integration Testing evaluates the interactions between different modules. It ensures that integrated components function as intended. Using Jest or Mocha in conjunction with tools like Supertest can facilitate thorough integration testing, providing confidence that the modules work together seamlessly.
End-to-End (E2E) Testing simulates real user scenarios to test the application flow from start to finish. Selenium is a widely-used tool for E2E testing, enabling developers to automate web browsers and verify that the application behaves as expected under various conditions. Selenium supports multiple programming languages, making it a versatile choice for developers.
For those looking to delve deeper into the intricacies of web testing, there are several comprehensive testing tutorials available. These resources can provide step-by-step guidance on implementing effective testing strategies, ensuring your web applications are robust and user-friendly.
By integrating these essential testing tools into your development workflow, you can significantly improve the quality and reliability of your web applications. Proper testing not only enhances performance but also builds user trust by delivering a seamless and bug-free experience.
Conclusion
In the rapidly evolving field of web development, equipping oneself with the right tools is indispensable for achieving efficiency and excellence. Throughout this blog post, we have highlighted ten essential tools that every web developer should consider integrating into their workflow. From code editors and version control systems to debugging tools and performance analyzers, each tool serves a unique purpose in streamlining the development process and enhancing overall productivity.
Using the appropriate tools can significantly reduce development time, minimize errors, and improve the quality of your code. Tools like Visual Studio Code and Git have become industry standards due to their robust features and ease of use. Similarly, leveraging task runners and package managers such as Gulp and npm can automate repetitive tasks, allowing developers to focus on more complex aspects of their projects.
We encourage you to explore the tools mentioned in this post and assess how they can be integrated into your current workflow to optimize your development practices. Adopting new tools may require an initial learning curve, but the long-term benefits far outweigh the effort. For further reading on optimizing your development process, consider exploring our related articles on choosing the right code editor and version control best practices.
By staying updated with the latest tools and technologies, web developers can not only enhance their skill set but also deliver high-quality web applications that meet modern standards. The right tools are not just accessories but essential components that contribute to the success of any web development project.